In today’s digital age, web applications have become a powerful medium for bringing traditional practices like astrology and numerology to a wider audience. These ancient arts of divination and self-discovery continue to fascinate people worldwide, and implementing them in an interactive web format offers both technical challenges and creative opportunities for developers.
In this comprehensive article, I’ll walk you through the initial development phase of an Astrology and Numerology web application. This first part will focus on building the foundation with HTML structure and implementing the core JavaScript functionality. By the end of this tutorial, you’ll understand how to create an interactive application that calculates and presents personalized astrological and numerological insights.
Project Overview
Our application consists of two main components:
- Astrology Game: A feature that calculates a user’s zodiac sign based on their birthdate and provides personalized predictions across various life aspects.
- Matrix of Destiny: A numerology calculator that creates a visual matrix representation of a person’s life path, soul number, and other numerological insights.
Let’s start by examining the HTML structure that forms the foundation of our application.
Read More : Github
HTML Structure Analysis
The application’s HTML structure follows modern web development practices, with clear semantic markup and a focus on user experience. Here’s a breakdown of the key elements:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Astrology & Numerology Game</title>
<link rel="stylesheet" href="/style/style.css">
</head>
<body>
<header>
<h1>Astrology & Numerology Game</h1>
<nav>
<button id="btnAstrology" class="active">Astrology Game</button>
<button id="btnNumerology">Matrix of Destiny</button>
</nav>
</header>
<main>
<!-- Astrology Section -->
<section id="astrologySection">
<!-- Content here -->
</section>
<!-- Numerology Section -->
<section id="numerologySection" class="display-none">
<!-- Content here -->
</section>
</main>
<script type="module" src="/script/script.js"></script>
</body>
</html>
The header includes a title and navigation buttons that switch between the two main features. The application uses the type="module"
attribute for JavaScript, indicating that we’re using ES6 modules for better code organization.
Astrology Section
The astrology section consists of a user input form and a results container:
<section id="astrologySection">
<div class="description">
<p>Discover your Zodiac sign, personality, and fate! Enter your details below to reveal your cosmic destiny.</p>
</div>
<div class="game-container">
<form id="astrology-form">
<div class="form-group">
<label for="astro-date">Enter your birthdate:</label>
<input type="date" id="astro-date" required>
</div>
<div class="form-group">
<label for="gender">Select Gender:</label>
<select id="gender" required>
<option value="">Choose...</option>
<option value="male">Male</option>
<option value="female">Female</option>
<option value="other">Other</option>
</select>
</div>
<div class="form-group">
<label for="phone-digit">Last Digit of Your Phone Number:</label>
<input type="number" id="phone-digit" min="0" max="9" required>
</div>
<button type="submit" class="submit-btn">Reveal Your Destiny</button>
</form>
<div class="results-container display-none">
<!-- Results content will be displayed here -->
</div>
</div>
</section>
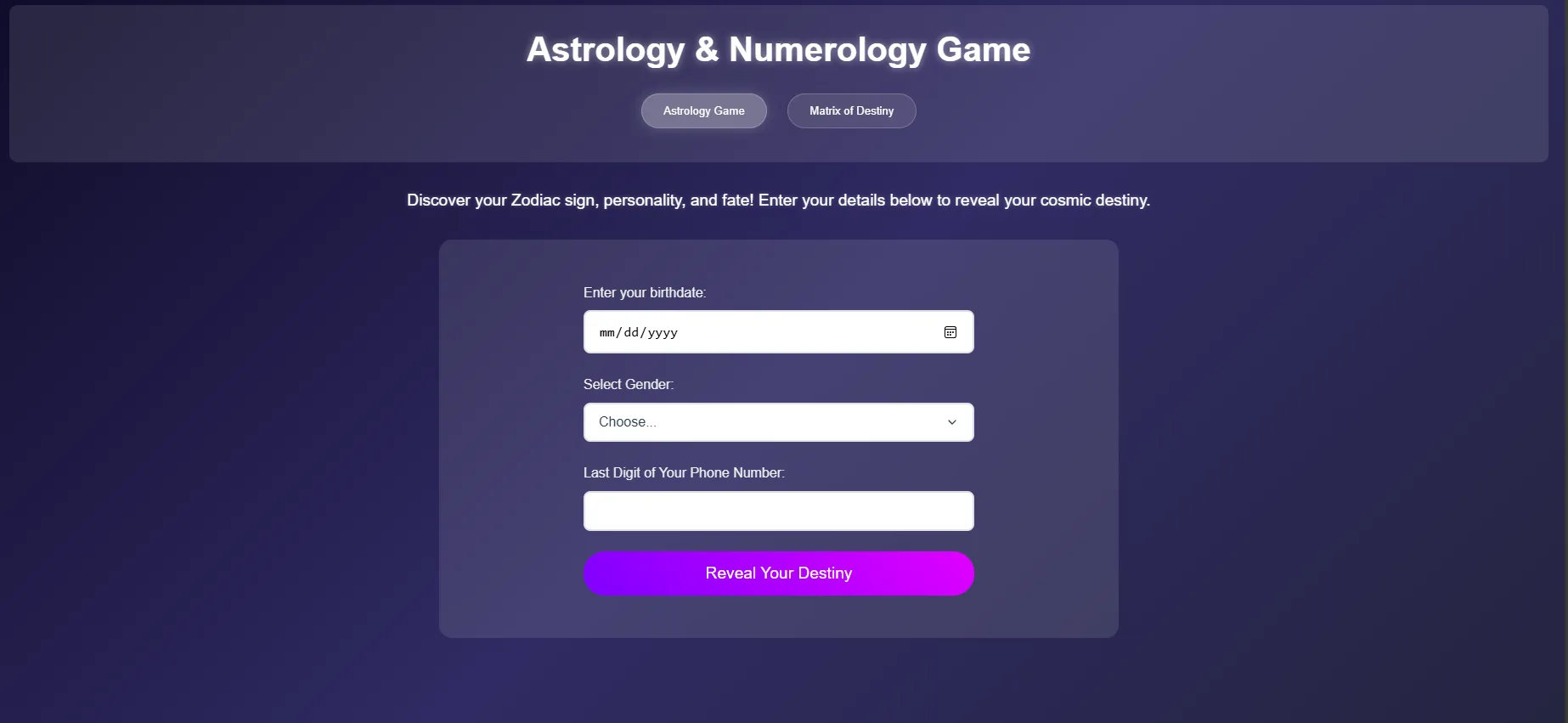
The form collects three key pieces of information:
- Birthdate (for zodiac sign calculation)
- Gender (for personalized predictions)
- Last digit of phone number (as an additional personalization factor)
The results container is initially hidden with the display-none
class and will be shown after form submission.
Numerology Section
The Matrix of Destiny section is similarly structured with a form and results container:
<section id="numerologySection" class="display-none">
<div class="description">
<p>Discover your Life Path, Soul Number, and Destiny Matrix through your birth date numerology.</p>
</div>
<div class="game-container">
<form id="numerology-form">
<div class="form-group">
<label for="num-date">Enter your birthdate:</label>
<input type="date" id="num-date" required>
</div>
<button type="submit" class="submit-btn">Calculate Matrix</button>
</form>
<div class="matrix-results display-none">
<h2>Matrix of Destiny</h2>
<div class="matrix-container">
<svg id="matrixSvg" width="100%" height="100%" version="1.1" viewBox="0 0 254 254" xmlns="http://www.w3.org/2000/svg">
<!-- SVG content will be dynamically generated -->
</svg>
</div>
<!-- Interpretation elements -->
</div>
</div>
</section>
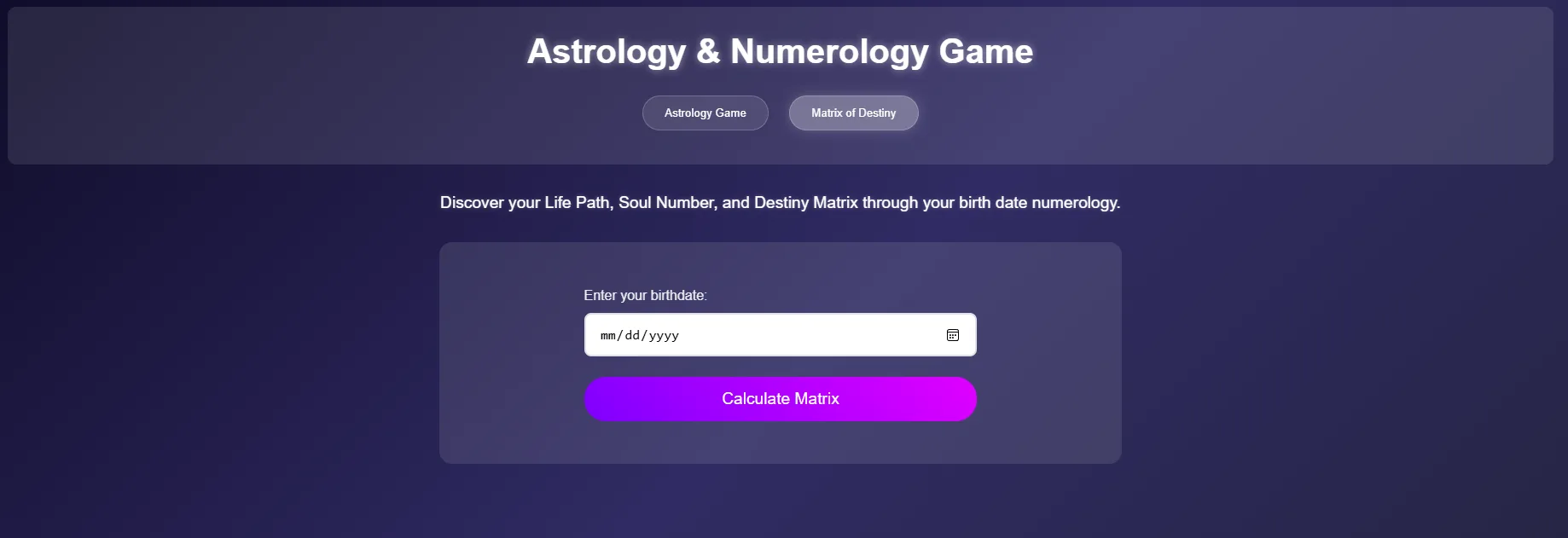
This section requires only the birthdate for calculation but includes a more complex results visualization using SVG for the matrix diagram and interpretation cards for different aspects of the numerological reading.
JavaScript Implementation
Now let’s examine the core JavaScript functionality that powers our application. The code is organized into modules for better maintainability:
script.js
: Main application logiczodiac.js
: Zodiac sign calculations and predictionsmatrix.js
: Matrix of Destiny calculations (to be covered in Part 2)
Main Script (script.js)
The main script acts as the controller for our application, handling UI interactions and connecting the various components:
// Import Matrix of Destiny
import { MatrixOfDestiny } from './matrix.js';
import { calculateZodiacSign, getRandomPrediction } from './zodiac.js';
// Store data for calculations
let matrixOfDestiny = null;
// DOM Elements
const btnAstrology = document.getElementById('btnAstrology');
const btnNumerology = document.getElementById('btnNumerology');
const astrologySection = document.getElementById('astrologySection');
const numerologySection = document.getElementById('numerologySection');
const numerologyForm = document.getElementById('numerology-form');
const astrologyForm = document.getElementById('astrology-form');
const matrixResults = document.querySelector('.matrix-results');
const matrixSvg = document.getElementById('matrixSvg');
// Initialize
document.addEventListener('DOMContentLoaded', () => {
// Set max date to today
const dateInputs = document.querySelectorAll('input[type="date"]');
const today = new Date().toISOString().split('T')[0];
dateInputs.forEach(input => {
input.max = today;
});
// Initialize Matrix of Destiny
matrixOfDestiny = new MatrixOfDestiny();
// Add event listeners
if (btnAstrology) btnAstrology.addEventListener('click', () => switchSection('astrology'));
if (btnNumerology) btnNumerology.addEventListener('click', () => switchSection('numerology'));
// Form submission handlers and other event listeners...
});
The initialization phase includes:
- Setting the maximum date for date inputs to prevent future dates
- Initializing the Matrix of Destiny component
- Adding event listeners for navigation and form submissions
Let’s continue with the form submission handlers and other core functions:
// Add astrology form submission handler
if (astrologyForm) {
astrologyForm.addEventListener('submit', (e) => {
e.preventDefault();
const birthdate = document.getElementById('astro-date').value;
const gender = document.getElementById('gender').value;
const phoneDigit = document.getElementById('phone-digit').value;
if (!birthdate || !gender || !phoneDigit) {
alert('Please fill in all fields');
return;
}
calculateAstrology(birthdate, gender, phoneDigit);
});
}
// Function to switch between sections
function switchSection(section) {
if (section === 'astrology') {
astrologySection.classList.remove('display-none');
numerologySection.classList.add('display-none');
btnAstrology.classList.add('active');
btnNumerology.classList.remove('active');
} else {
numerologySection.classList.remove('display-none');
astrologySection.classList.add('display-none');
btnNumerology.classList.add('active');
btnAstrology.classList.remove('active');
}
}
The switchSection
function handles the UI toggling between astrology and numerology sections, providing a seamless user experience when navigating the application.

Astrology Calculation
The calculateAstrology
function processes the user’s input and displays the results:
// Function to calculate astrology
function calculateAstrology(birthdate, gender, phoneDigit) {
const zodiacSign = calculateZodiacSign(birthdate);
// Display results container
const resultsContainer = document.querySelector('.results-container');
resultsContainer.classList.remove('display-none');
// Update zodiac symbol and traits
const symbolElement = document.querySelector('.zodiac-symbol');
const traitsElement = document.querySelector('.zodiac-traits');
symbolElement.textContent = zodiacSign.symbol;
traitsElement.innerHTML = `
<h3>${zodiacSign.name}</h3>
<p><strong>Element:</strong> <span class="element-${zodiacSign.element.toLowerCase()}">${zodiacSign.element}</span></p>
<p><strong>Quality:</strong> <span class="quality-badge quality-${zodiacSign.quality.toLowerCase()}">${zodiacSign.quality}</span></p>
<p><strong>Ruler:</strong> ${zodiacSign.ruler}</p>
<p><strong>Personality:</strong> ${zodiacSign.traits.personality}</p>
<p><strong>Strengths:</strong> ${zodiacSign.traits.strengths}</p>
<p><strong>Lucky Numbers:</strong> ${zodiacSign.traits.luckyNumbers.join(', ')}</p>
<p><strong>Lucky Colors:</strong> ${zodiacSign.traits.luckyColors.join(', ')}</p>
`;
// Show initial prediction for love category
updatePrediction('love', zodiacSign);
}
This function:
- Gets the zodiac sign information by calling
calculateZodiacSign
from the zodiac module - Makes the results container visible
- Updates the UI with the zodiac symbol and traits
- Shows an initial prediction for the “love” category
Prediction Updates
The application allows users to explore different prediction categories. Here’s how the prediction updating works:
// Function to update prediction
function updatePrediction(category, zodiacSign = null) {
if (!zodiacSign) {
const birthdate = document.getElementById('astro-date').value;
zodiacSign = calculateZodiacSign(birthdate);
}
// Update active category
const categories = document.querySelectorAll('.category');
categories.forEach(cat => {
cat.classList.remove('active');
if (cat.dataset.category === category) {
cat.classList.add('active');
}
});
// Get and display prediction
const prediction = getRandomPrediction(zodiacSign, category);
const predictionContent = document.querySelector('.prediction-content');
predictionContent.innerHTML = `<p class="prediction-text">${prediction}</p>`;
}
When a user clicks on a prediction category (love, career, money, etc.), this function:
- Updates the active category in the UI
- Gets a random prediction for that category
- Updates the prediction content display
Clear Results Function
To enhance user experience, the application includes a clear function to reset the forms and results:
// Function to clear results
function clearResults(sectionId) {
if (sectionId === 'astrologySection') {
// Clear astrology form and results
document.getElementById('astro-date').value = '';
document.getElementById('gender').value = '';
document.getElementById('phone-digit').value = '';
const resultsContainer = document.querySelector('.results-container');
if (resultsContainer) {
resultsContainer.classList.add('display-none');
}
document.querySelector('.zodiac-symbol').textContent = '';
document.querySelector('.zodiac-traits').innerHTML = '';
document.querySelector('.prediction-content').innerHTML = '';
document.querySelectorAll('.category').forEach(cat => cat.classList.remove('active'));
} else if (sectionId === 'numerologySection') {
// Clear numerology form and results
document.getElementById('num-date').value = '';
if (matrixResults) {
matrixResults.classList.add('display-none');
}
if (matrixSvg) {
matrixSvg.innerHTML = '';
}
const interpretationDisplay = document.querySelector('.interpretation-display');
if (interpretationDisplay) {
interpretationDisplay.innerHTML = '';
}
document.querySelectorAll('.interpretation-cards .card').forEach(card => {
card.classList.remove('active');
});
}
}
Zodiac Sign Implementation (zodiac.js)
The zodiac.js module contains the data and logic for the astrology feature. Let’s examine its core components:
Zodiac Signs Data Structure
The module defines a comprehensive data structure for zodiac signs:
export const zodiacSigns = {
aries: {
name: 'Aries',
symbol: '♈',
startDate: { month: 3, day: 21 },
endDate: { month: 4, day: 19 },
element: 'Fire',
quality: 'Cardinal',
ruler: 'Mars',
traits: {
personality: 'Confident, courageous, enthusiastic, impulsive, energetic',
strengths: 'Natural leader, determined, optimistic',
challenges: 'Impatience, short temper, impulsiveness',
compatibility: ['Leo', 'Sagittarius', 'Gemini', 'Aquarius'],
luckyNumbers: [1, 9, 8],
luckyColors: ['Red', 'Orange'],
predictions: {
love: [
'Your bold nature will attract an exciting new relationship',
'Take initiative in matters of the heart',
'Your passion will lead to meaningful connections',
'A surprising romantic encounter awaits you',
'Trust your instincts in relationship matters'
],
money: [
// Money predictions...
],
career: [
// Career predictions...
],
life: [
// Life predictions...
],
health: [
// Health predictions...
]
}
}
},
// Other zodiac signs...
};
Each zodiac sign contains:
- Basic information (name, symbol, date range)
- Astrological attributes (element, quality, ruler)
- Detailed personality traits
- Arrays of predictions for different life categories
Zodiac Calculation Function
The core calculation function determines a person’s zodiac sign based on their birthdate:
// Enhanced zodiac calculation function
function calculateZodiacSign(birthdate) {
const date = new Date(birthdate);
const month = date.getMonth() + 1;
const day = date.getDate();
function isDateInRange(date, sign) {
const { startDate, endDate } = sign;
if (startDate.month === 12 && endDate.month === 1) {
return (month === 12 && day >= startDate.day) ||
(month === 1 && day <= endDate.day);
}
if (month === startDate.month) {
return day >= startDate.day;
} else if (month === endDate.month) {
return day <= endDate.day;
}
return month > startDate.month && month < endDate.month;
}
for (const [key, sign] of Object.entries(zodiacSigns)) {
if (isDateInRange(date, sign)) {
return sign;
}
}
return zodiacSigns.capricorn;
}
This function works by:
- Extracting the month and day from the user’s birthdate
- Using the
isDateInRange
helper function to check if the date falls within each zodiac sign’s range - Handling the special case of Capricorn, which spans December and January
- Returning the matching zodiac sign object
Prediction Generation
The module also includes a function to generate personalized predictions:
// Enhanced prediction system
function getRandomPrediction(sign, category) {
const predictions = sign.traits.predictions[category];
const randomIndex = Math.floor(Math.random() * predictions.length);
return predictions[randomIndex];
}
This function selects a random prediction from the appropriate category for the user’s zodiac sign, adding an element of variation to the user experience.
Interactive Features
The application includes several interactive elements that enhance user engagement:
// Add event listeners for prediction categories
document.querySelectorAll('.category').forEach(category => {
category.addEventListener('click', () => {
const categoryType = category.dataset.category;
updatePrediction(categoryType);
});
});
// Add event listener for "Try Again" button
document.querySelector('.try-again').addEventListener('click', () => {
const activeCategory = document.querySelector('.category.active');
if (activeCategory) {
updatePrediction(activeCategory.dataset.category);
}
});
// Add event listener for "Reset" button
document.querySelector('.reset-btn').addEventListener('click', () => {
clearResults('astrologySection');
});
These event listeners allow users to:
- Switch between different prediction categories
- Get a new prediction for the current category
- Reset the form and start over
Responsive Design Considerations
The application’s HTML structure includes responsive design elements:
<div class="predictions">
<h2>Your Predictions</h2>
<div class="prediction-categories">
<div class="category" data-category="love">Love</div>
<div class="category" data-category="money">Money</div>
<div class="category" data-category="career">Career</div>
<div class="category" data-category="life">Life</div>
<div class="category" data-category="health">Health</div>
</div>
<div class="prediction-content"></div>
</div>
<div class="action-buttons">
<button class="print-btn">Print Results</button>
<button class="clear-btn">Clear</button>
</div>
</div>
</div>
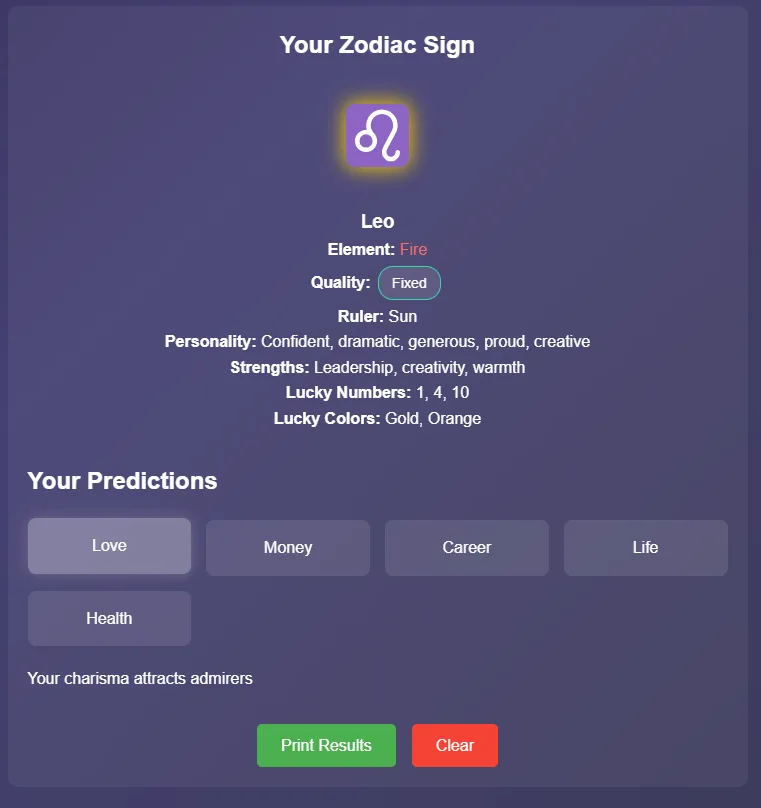
The categories are displayed with text, making them easily accessible on both desktop and mobile devices. The prediction display area is designed to adapt to different screen sizes, ensuring a good user experience across devices.
Conclusion and Next Steps
In this first part of our tutorial, we’ve covered the fundamental structure and functionality of our Astrology and Numerology web application. We’ve implemented:
- The basic HTML structure for both the Astrology Game and Matrix of Destiny features
- The JavaScript functionality for calculating zodiac signs and generating predictions
- Interactive elements for user engagement
- Responsive design considerations
In Part 2, we’ll focus on:
- Implementing the Matrix of Destiny numerology feature
- Creating the SVG visualization for the numerology matrix
- Adding CSS styling to enhance the visual appeal
- Implementing additional interactive features and animations
This foundation provides a solid starting point for building an engaging and interactive web application that brings the ancient practices of astrology and numerology into the digital age.