Introduction to HTML
HTML (HyperText Markup Language) is the foundation of web development. It provides the structure and organization for web pages, allowing browsers to interpret and display content correctly. HTML is not a programming language but rather a markup language that defines elements using tags.
Below is a simple HTML structure:
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
</head>
<body>
<h1>My First Heading</h1>
<p>My First Paragraph.</p>
</body>
</html>
Understanding HyperText and Markup Language
What is HyperText?
HyperText refers to a system where text and multimedia elements (such as images, videos, and buttons) can be interconnected via hyperlinks. This allows users to navigate between different pieces of content in a non-linear fashion.
What is a Markup Language?
A markup language is a system of symbols inserted into a document to define its structure and formatting. These symbols, or “tags,” do not appear in the final output but provide instructions to the browser on how to display content.
HTML Elements and Structure
An HTML element consists of:
- A start tag:
<tagname>
- Content: The actual data inside the element.
- An end tag:
</tagname>
<p>This is a paragraph.</p>
<p>
→ Start tagThis is a paragraph.
→ Content</p>
→ End tag
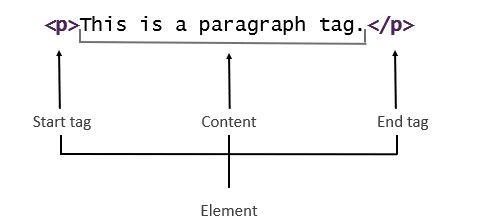
HTML Document Structure
Every HTML document follows a specific structure, starting with the <!DOCTYPE>
declaration:
1. <!DOCTYPE>
Declaration
The <!DOCTYPE>
declaration is not an HTML tag but an instruction that tells the browser which version of HTML the document follows.
For HTML5:
<!DOCTYPE html>
Older versions:
HTML 4.01:
<code><!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"></code>
XHTML 1.1:
<code><!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.1//EN" "http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd"></code>
2. The <html>
Tag
The <html>
element is the root of an HTML document. All other elements must be placed inside it.
Example:
<html lang="en">
</html>
The lang="en"
attribute specifies the language of the document, improving accessibility for screen readers.
3. The <head>
Section
The <head>
element contains metadata, which is information about the webpage that isn’t displayed directly.
Important elements in <head>
:
- Title: Sets the page title in the browser tab.
- Meta Tags: Define character encoding, viewport settings, and other metadata.
- CSS and JavaScript Links: Connect external stylesheets and scripts.
Tags are allowed in the <head>:
<base>,
<link>,
<meta>,
<script>,
<noscript>,
<style>,
<title>
Example:
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My Webpage</title>
</head>
Anything inside <head>
element is not going to be displayed
The <head> element is a container for metadata
<title>

The text within the <title>
is visible at the top of any web page in the browser’s title bar.
4. The <body>
Section
The <body>
contains the main content of the webpage, such as text, images, and links.
Example:
<body>
<h1>Welcome to My Website</h1>
<p>This is a sample paragraph.</p>
</body>
HTML Headings and Text Elements
HTML includes six levels of headings, from <h1>
(largest and most important) to <h6>
(smallest and least important).
<h1>Main Title</h1>
<h2>Section Heading</h2>
<h3>Subsection Heading</h3>
<h4>Minor Section</h4>
<h5>Smaller Heading</h5>
<h6>Least Important Heading</h6>
Text Formatting Elements
- Bold:
<b>
or<strong>
(important text) - Italic:
<i>
or<em>
(emphasized text) - Underline:
<u>
- Strikethrough:
<s>
- Small text:
<small>
<p>This is <b>bold</b>, <i>italic</i>, and <u>underlined</u>.</p>
HTML Attributes
Attributes provide additional information about elements.
Example:
<p class="intro">This is a paragraph with a class attribute.</p>
class="intro"
→ Assigns a CSS class to the paragraph.
Common Attributes
id
: Unique identifier for an element.class
: Assigns an element to a CSS class.href
: Specifies a link’s destination in<a>
tags.src
: Defines the source of an image or other media.alt
: Provides alternative text for images.title
: Displays a tooltip when hovering over an element.
Example:
<a href="https://www.google.com">Go to Google</a>
<img src="image.jpg" alt="Description of image">
HTML Links and Navigation
Hyperlinks are created using the <a>
(anchor) tag.
Example:
<a href="https://www.example.com">Visit Example</a>
You can also link to specific sections of a page using id
attributes:
<a href="#section2">Jump to Section 2</a>
<h2 id="section2">Section 2</h2>
Void Elements (Self-Closing Tags)
Some HTML elements do not require a closing tag. These include:
<img>
: Images<br>
: Line breaks<hr>
: Horizontal lines<input>
: Form input fields<meta>
: Metadata elements
Example:
<img src="image.jpg" alt="An image">
<br>
<hr>
Semantic HTML
Semantic elements provide meaningful structure to a webpage. Unlike <div>
, which is a generic container, semantic elements define their purpose.
Common Semantic Elements
<header>
: Defines introductory content, often includes navigation.
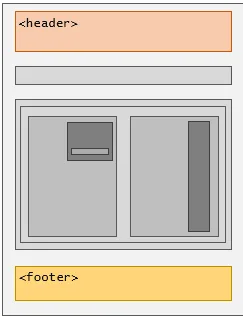
<nav>
: Contains main navigation links.
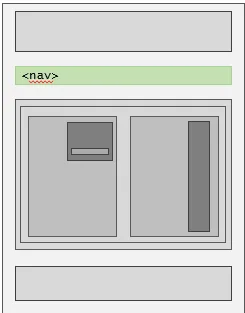
<main>
: Wraps the main content of the page.
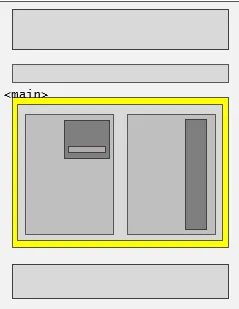
<section>
: Groups related content.
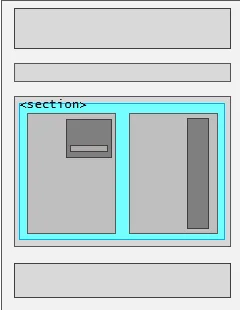
<article>
: Defines self-contained content like blog posts or news articles.
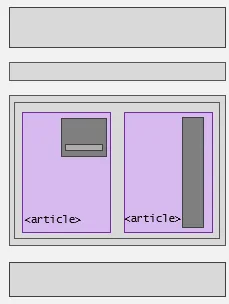
<aside>
: Represents secondary content like sidebars.
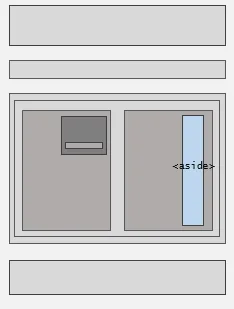
<footer>
: Contains copyright information and additional links.
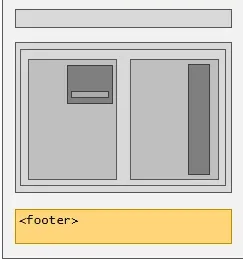
<figure>
and<figcaption>
: To wrap images with text, you can use the<figure>
and<figcaption>
elements. However,<figure>
elements can contain all types of content, including text, images, tables, audio, and video<figcaption>
elements provide captions to figures
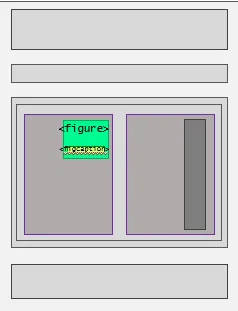
Noted: Remember <article> and <section> are for grouping content that is related in some way
A <div> is just the most generic container that contains a variety of content
<div> elements are still needed in HTML5!
Example:
<header>
<h1>Website Title</h1>
<nav>
<a href="home.html">Home</a>
<a href="about.html">About</a>
</nav>
</header>
<main>
<article>
<h2>Article Title</h2>
<p>This is an article about HTML.</p>
</article>
</main>
<footer>
<p>© 2025 My Website</p>
</footer>
Using semantic HTML—which involves using HTML tags that clearly describe their purpose—offers several advantages:
- Improved Search Engine Optimization (SEO): Search engines analyze web pages to determine their content and relevance. By using semantic tags, you help search engines understand the structure and importance of your content, which can positively influence your site’s search rankings.
- Enhanced Accessibility: Screen readers and other assistive technologies rely on semantic HTML to navigate and interpret web content. Using appropriate tags ensures that visually impaired users can effectively understand and interact with your site.
- Easier Code Maintenance: Semantic HTML provides a clear structure to your code, making it more readable and maintainable. This clarity simplifies the process of updating and managing your codebase, as developers can easily identify and work with different sections of the page.
Additional HTML Concepts
Comments in HTML
Comments help document code and are ignored by browsers.
<!-- This is a comment -->
Favicon
A favicon is a small icon displayed in browser tabs.
<link rel="icon" type="image/png" href="favicon.png">
HTML Boilerplate (Quick Setup)
If using VSCode, type !
and press Tab
to generate a basic HTML boilerplate.
Conclusion
HTML is the cornerstone of web development, enabling the creation of structured, accessible, and interactive web pages. By mastering its elements, attributes, and semantic structure, developers can build user-friendly and efficient websites.
To continue learning, check out our next guide: Creating a Web Portfolio and Deploying to GitHub.