In our previous article, we laid the groundwork for our Matrix of Destiny web application, introducing the concept and establishing our development approach. Now we’re diving deeper into implementation, focusing on bringing the numerology matrix to life through JavaScript, SVG, and CSS. This part explores how we transform mathematical principles into an interactive visual experience that users can engage with meaningfully.
Understanding the Matrix of Destiny
The Matrix of Destiny is an advanced numerological system that maps various aspects of a person’s life based on their birth date. Unlike simpler numerology systems that calculate only a few numbers, this matrix creates an interconnected web of values representing different areas of life: core essence, relationships, material path, karmic lessons, and hidden talents, among others.
The beauty of this system lies in how these numbers interact, forming patterns that reveal deeper insights about one’s life journey. Our goal is to create a digital representation that maintains this interconnectedness while making the information accessible and visually appealing.
Core Architecture of the Matrix Visualization
The heart of our application is the Matrix Of Destiny class defined in matrix.js. This class is responsible for calculating numerological values and rendering them as an interactive SVG visualization. Let’s examine its structure and key functionality.
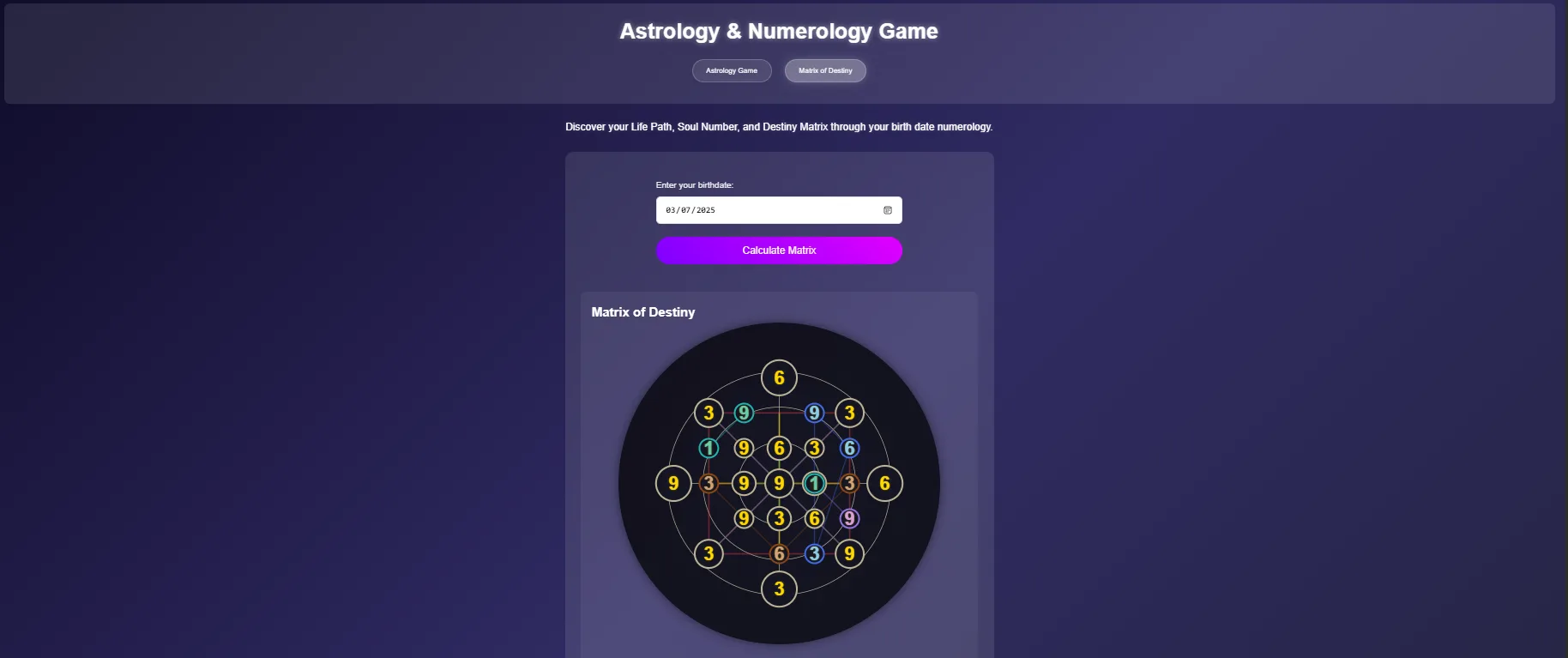
Class Structure Overview
The Matrix Of Destiny class encapsulates several key responsibilities:
- Initializing and managing the SVG canvas
- Calculating numerological values from birth dates
- Rendering the matrix diagram with position nodes and connection lines
- Handling user interactions like hovering and clicking
- Providing interpretations for different aspects of the matrix
The class is designed with a clean separation of concerns, making it maintainable and extensible as the application grows.
Initializing the SVG Canvas
The first step in creating our matrix visualization is establishing the SVG structure:
initializeMatrix() {
const svg = document.getElementById('matrixSvg');
if (!svg) return;
// Set viewBox and other SVG attributes
svg.setAttribute('viewBox', '-10 -10 274 274');
svg.setAttribute('width', '100%');
svg.setAttribute('height', '100%');
svg.setAttribute('preserveAspectRatio', 'xMidYMid meet');
// Clear existing content
svg.innerHTML = '';
// Create layers
const layer1 = this.createLayer('layer1');
const layer2 = this.createLayer('layer2');
const layer3 = this.createLayer('layer3');
const layer4 = this.createLayer('layer4');
svg.appendChild(layer1);
svg.appendChild(layer2);
svg.appendChild(layer3);
svg.appendChild(layer4);
matrixLayer = layer3; // Main layer for dynamic content
this.drawBaseMatrix();
}
This method configures the SVG canvas with appropriate dimensions and creates a layered structure. The layering approach is crucial for maintaining a clean visual hierarchy:
- Layer 1: Base matrix circle and foundational grid lines
- Layer 2: Background elements
- Layer 3: Interactive matrix elements including nodes and connection lines
- Layer 4: Overlay elements like tooltips and highlights
Using layers allows us to manage complex SVG elements more effectively, adjusting opacity or applying effects to specific groups without affecting others.
Drawing the Foundation
The base matrix structure consists of concentric circles and connecting lines:
drawBaseMatrix() {
const layer1 = document.querySelector('.layer1');
if (!layer1) return;
// Set base styles for layer1
layer1.setAttributeNS(null, 'stroke', '#bcb395');
layer1.setAttributeNS(null, 'stroke-width', '0.5');
// Add outer circle
const outerCircle = document.createElementNS(svgNS, "circle");
outerCircle.setAttributeNS(null, "cx", "127");
outerCircle.setAttributeNS(null, "cy", "127");
outerCircle.setAttributeNS(null, "r", "95");
outerCircle.setAttributeNS(null, "fill", "none");
outerCircle.setAttributeNS(null, "stroke", "#bcb395");
outerCircle.setAttributeNS(null, "stroke-width", "0.5");
layer1.appendChild(outerCircle);
// Additional circles and lines...
}
This creates the framework upon which our numerological values will be placed. The concentric circles represent different spheres of influence in a person’s life, while the connecting lines show how these spheres interact with each other.
Calculating Numerological Values
The core of any numerology application is its calculation engine. Our calculate
method processes a birth date and derives the fundamental numbers for our matrix:
calculate(birthdate) {
const date = new Date(birthdate);
const day = date.getDate();
const month = date.getMonth() + 1;
const year = date.getFullYear();
// Calculate core numbers
const core = this.calculateSingleDigit(day + month + this.calculateSingleDigit(year));
const portrait = this.calculateSingleDigit(day);
const karmic = this.calculateSingleDigit(month);
const relationship = this.calculateSingleDigit(year);
const money = this.calculateSingleDigit(core + portrait);
const talents = this.calculateSingleDigit(karmic + relationship);
return {
core,
portrait,
karmic,
relationship,
money,
talents,
day,
month,
year
};
}
The helper method calculateSingleDigit
is fundamental to numerology calculations, reducing multi-digit numbers to a single digit (1-9):
calculateSingleDigit(num) {
if (typeof num !== 'number') {
num = parseInt(num);
}
if (isNaN(num)) return 0;
if (num <= 9) return num;
const sum = String(num).split('').reduce((a, b) => parseInt(a) + parseInt(b), 0);
if (sum <= 9) return sum;
return this.calculateSingleDigit(sum);
}
This recursive approach continues adding digits until a single digit is achieved, embodying the numerological principle that all numbers can be reduced to their essence.
The Visual Structure of the Matrix
Our matrix design places numerological values in specific positions, each representing different aspects of life. The positions
array defines these locations:
this.positions = [
// Core position (Center)
{
x: 127, y: 127,
value: numbers.core,
label: 'Core Essence',
class: 'center',
type: 'core',
radius: 12,
description: 'Your soul essence and life purpose',
connectedPaths: ['core-path']
},
// Outer positions (Cardinal Points)
{
x: 127, y: 37,
value: numbers.portrait,
label: 'Self Image',
class: 'outer-north',
type: 'portrait',
radius: 15,
description: 'How others perceive you',
connectedPaths: ['core-path', 'material-path']
},
// More positions...
];
Each position includes:
- Coordinates (
x
,y
) for placement - The numerological
value
to display - A
label
explaining what this position represents - CSS
class
for styling - A
type
identifier for interpretations - Size (
radius
) for visual hierarchy - A
description
for tooltips connectedPaths
showing relationships to other positions
This structured approach makes it easy to maintain consistent positioning while allowing visual customization through CSS.
Interactive Elements and User Experience
To make the matrix truly engaging, we add interactive elements like hover effects and tooltips:
handleMouseOver = (e, circle, number, label, pos, tooltip) => {
// Only show tooltip, no movement or scaling
tooltip.textContent = `${pos.label}: ${pos.description}`;
tooltip.style.left = `${e.pageX + 15}px`;
tooltip.style.top = `${e.pageY + 15}px`;
tooltip.classList.add('visible');
// Highlight connected paths
if (pos.connectedPaths) {
pos.connectedPaths.forEach(pathClass => {
const path = document.querySelector(`.${pathClass}`);
if (path) {
path.style.opacity = '0.8';
}
});
}
};
This creates a dynamic experience where users can explore the matrix intuitively. When hovering over a position, related paths are highlighted, visually demonstrating the interconnectedness of different life aspects.
For a deeper level of interaction, we implement click events that display detailed interpretations:
group.addEventListener('click', () => {
document.querySelectorAll('.interpretation-cards .card').forEach(card => {
card.classList.remove('active');
});
const card = document.querySelector(`.interpretation-cards .card[data-type="${pos.type}"]`);
if (card) {
card.classList.add('active');
this.showInterpretation(pos.type, this.getMatrixMeaning(pos.type, pos.value));
}
});
The interpretation system maps each number (1-9) to specific meanings for different aspects of life:
getMatrixMeaning(type, number) {
const meanings = {
core: {
1: "You are a natural born leader with a pioneering spirit. Your life path is marked by independence, originality, and innovation...",
// Other interpretations...
},
// Other types...
};
return meanings[type]?.[number] || "Seek deeper meaning in your numbers...";
}
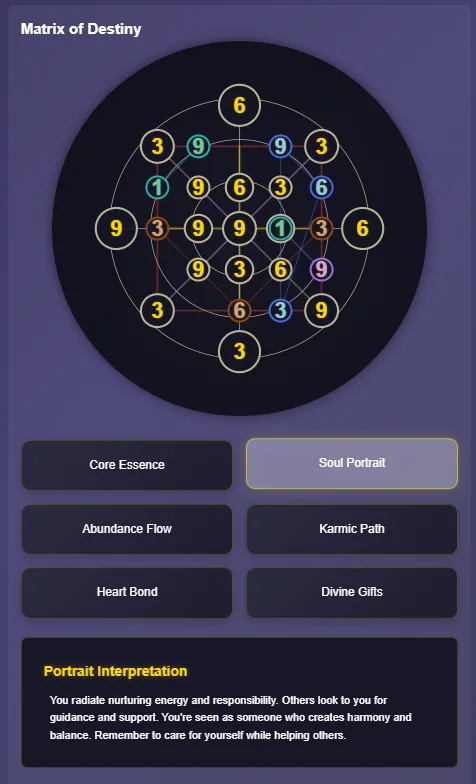
This comprehensive database of interpretations brings depth to the user experience, providing personalized insights based on their unique matrix.
Visual Connections: The Web of Life
Perhaps the most visually striking feature of our matrix is the network of connection lines linking different positions:
addConnectionLines() {
// Create connection lines layer
const linesLayer = document.createElementNS(svgNS, "g");
linesLayer.setAttributeNS(null, "class", "connection-lines");
// Define connection paths with meaningful names and colors
const connections = [
// Main Cross (Core to Cardinal Points)
{
path: `M 127,127 L 127,67
M 127,127 L 187,127
M 127,127 L 127,187
M 127,127 L 67,127`,
class: "core-path",
color: "#ffd700",
width: "1.5"
},
// Other connections...
];
// Add paths with enhanced styling
connections.forEach(conn => {
const path = document.createElementNS(svgNS, "path");
path.setAttributeNS(null, "d", conn.path);
path.setAttributeNS(null, "class", `connection-line ${conn.class}`);
path.setAttributeNS(null, "stroke", conn.color);
path.setAttributeNS(null, "stroke-width", conn.width);
path.setAttributeNS(null, "fill", "none");
path.setAttributeNS(null, "stroke-linecap", "round");
path.setAttributeNS(null, "stroke-linejoin", "round");
// Add animation for path drawing
const length = path.getTotalLength();
path.style.strokeDasharray = length;
path.style.strokeDashoffset = length;
path.style.animation = `drawPath 1.5s ease-out forwards`;
linesLayer.appendChild(path);
});
// Add lines layer before the circles layer
matrixLayer.insertBefore(linesLayer, matrixLayer.firstChild);
}
Each connection type has distinct styling, using color and stroke width to convey different types of relationships:
- Gold for core paths (radiating from the center)
- Red for the material world connections
- Green for inner world connections
- Purple for spiritual paths
The animated drawing effect (using SVG stroke animations) gives the matrix a sense of organic growth, emerging before the user’s eyes when they first calculate their numbers.
Enhancing the Visual Experience with CSS
While our matrix.js file creates the structure, the style.css file brings it to life visually. Let’s explore some key styling approaches:
/* Matrix diagram */
.matrix-container {
position: relative;
width: 100%;
max-width: 600px;
margin: 0 auto;
overflow: visible;
}
#matrixSvg {
background: radial-gradient(circle at center, rgba(20, 20, 35, 0.98), rgba(15, 15, 25, 0.99));
border-radius: 50%;
box-shadow: 0 0 20px rgba(0, 0, 0, 0.5);
}
.matrix-position {
fill: rgba(20, 20, 35, 0.95);
stroke: #bcb395;
stroke-width: 1.5;
}
.matrix-number {
fill: #ffd700;
font-size: 16px;
font-weight: bold;
text-shadow: 0 0 3px rgba(255, 215, 0, 0.5);
}
.matrix-label {
display: none;
}
.connection-line {
opacity: 0.4;
pointer-events: none;
}
/* Path colors */
.core-path { stroke: #ffd700; }
.material-path { stroke: #E54144; }
.inner-path { stroke: #009E52; }
.spiritual-path { stroke: #664074; }
.cycle-path { stroke: #4B0082; stroke-dasharray: 4; }
.challenge-path { stroke: #8B4513; stroke-dasharray: 3 2; }
.pinnacle-path { stroke: #4169E1; stroke-dasharray: 5 3; }
.personal-path { stroke: #20B2AA; stroke-dasharray: 2 2; }
.universal-path { stroke: #9370DB; stroke-dasharray: 4 2; }
/* Position type-specific styles */
[class*="challenge-"] .matrix-position { stroke: #8B4513; }
[class*="pinnacle-"] .matrix-position { stroke: #4169E1; }
[class*="personal-"] .matrix-position,
[class*="next-"] .matrix-position { stroke: #20B2AA; }
[class*="universal-"] .matrix-position { stroke: #9370DB; }
/* Number colors */
[class*="challenge-"] .matrix-number { fill: #d4a276; }
[class*="pinnacle-"] .matrix-number { fill: #87ceeb; }
[class*="personal-"] .matrix-number,
[class*="next-"] .matrix-number { fill: #66cdaa; }
[class*="universal-"] .matrix-number { fill: #dda0dd; }
/* Matrix tooltip */
.matrix-tooltip {
position: absolute;
display: none;
background: rgba(20, 20, 35, 0.95);
color: #bcb395;
padding: 8px 12px;
border-radius: 4px;
font-size: 12px;
max-width: 200px;
z-index: 1000;
box-shadow: 0 2px 8px rgba(0, 0, 0, 0.3);
pointer-events: none;
}
.matrix-tooltip.visible {
display: block;
}
The CSS creates a cosmic, mystical atmosphere appropriate for a numerology application, with a dark background and glowing elements. The radial gradient background suggests energy emanating from the center, while subtle box shadows and glows create depth and dimension.
Position-specific styling uses color coding to help users understand the meaning and relationships between different areas of the matrix. The animation keyframes for drawing paths add a dynamic feel to the visualization process.
Main Script Integration and User Flow
To connect our matrix visualization with the broader application, we need to integrate it with our main Script.js file:
// Initialize Matrix of Destiny
matrixOfDestiny = new MatrixOfDestiny();
// Add event listeners
if (btnAstrology) btnAstrology.addEventListener('click', () => switchSection('astrology'));
if (btnNumerology) btnNumerology.addEventListener('click', () => switchSection('numerology'));
if (numerologyForm) {
numerologyForm.addEventListener('submit', (e) => {
e.preventDefault();
const birthdate = document.getElementById('num-date').value;
if (!birthdate) {
alert('Please enter your birthdate');
return;
}
calculateNumerology(birthdate);
});
}
// Calculate numerology
function calculateNumerology(birthdate) {
if (!matrixOfDestiny) {
matrixOfDestiny = new MatrixOfDestiny();
}
const numbers = matrixOfDestiny.calculate(birthdate);
if (matrixResults && matrixSvg) {
matrixResults.classList.remove('display-none');
matrixOfDestiny.displayMatrixDiagram(numbers);
// Add click events for interpretation cards after matrix is displayed
document.querySelectorAll('.interpretation-cards .card').forEach(card => {
const type = card.getAttribute('data-type');
const position = matrixOfDestiny.positions.find(pos => pos.type === type);
if (position) {
card.addEventListener('click', () => {
document.querySelectorAll('.interpretation-cards .card').forEach(c => {
c.classList.remove('active');
});
card.classList.add('active');
matrixOfDestiny.showInterpretation(type, matrixOfDestiny.getMatrixMeaning(type, position.value));
});
}
});
}
return numbers;
}
This script handles the overall application flow, including:
- Initializing the matrix when the page loads
- Processing user input (birth date)
- Triggering calculations and displaying the matrix
- Managing theme preferences with localStorage
- Setting up universal tooltips for UI elements
The Script.js file serves as the controller in our MVC-like architecture, coordinating between user inputs and the matrix visualization.
Responsive Design Considerations
For optimal user experience across devices, our CSS includes responsive design principles:
/* Responsive adjustments */
@media (max-width: 768px) {
.matrix-container {
max-width: 90vw;
}
.interpretation-cards {
flex-direction: column;
}
.interpretation-card {
width: 100%;
margin: 5px 0;
}
.matrix-results-tables {
flex-direction: column;
}
.matrix-table {
width: 100%;
margin: 10px 0;
}
.matrix-label {
display: none;
}
}
@media (max-width: 480px) {
.matrix-number {
font-size: 10px;
}
.interpretation-result {
padding: 10px;
}
.birth-input-container {
flex-direction: column;
}
#birthdate, #calculate-matrix {
width: 100%;
margin: 5px 0;
}
}
These media queries ensure the application remains usable on tablets and smartphones, adapting the layout and simplifying certain elements when necessary. On smaller screens, we hide the position labels to reduce clutter but maintain the essential numerical values.
Animation and Visual Feedback
To make the application feel alive and responsive, we incorporate animations for various interactions:
@keyframes pulseGlow {
0% {
text-shadow: 0 0 10px rgba(255, 215, 0, 0.5);
}
50% {
text-shadow: 0 0 20px rgba(255, 215, 0, 0.8),
0 0 30px rgba(255, 215, 0, 0.4);
}
100% {
text-shadow: 0 0 10px rgba(255, 215, 0, 0.5);
}
}
/* Element colors */
.element-fire { color: #ff6b6b; }
.element-earth { color: #38d9a9; }
.element-air { color: #74c0fc; }
.element-water { color: #748ffc; }
/* Quality badges */
.quality-badge {
display: inline-block;
padding: 0.3rem 0.8rem;
border-radius: 15px;
font-size: 0.9rem;
margin: 0.2rem;
background: rgba(255, 255, 255, 0.1);
border: 1px solid rgba(255, 255, 255, 0.2);
}
.quality-cardinal { border-color: #ff6b6b; }
.quality-fixed { border-color: #38d9a9; }
.quality-mutable { border-color: #74c0fc; }
/* Prediction cards */
.prediction-card {
background: rgba(255, 255, 255, 0.05);
border-radius: 10px;
padding: 1.5rem;
margin: 1rem 0;
transition: all 0.3s ease;
border: 1px solid rgba(255, 255, 255, 0.1);
}
.prediction-card:hover {
background: rgba(255, 255, 255, 0.1);
transform: scale(1.02);
border-color: rgba(255, 255, 255, 0.2);
}
/* Lucky elements */
.lucky-section {
display: flex;
gap: 1rem;
flex-wrap: wrap;
margin: 1rem 0;
}
.lucky-item {
background: rgba(255, 255, 255, 0.05);
border-radius: 20px;
padding: 0.5rem 1rem;
display: flex;
align-items: center;
gap: 0.5rem;
}
.lucky-number {
color: #ffd700;
font-weight: bold;
}
.lucky-color {
width: 15px;
height: 15px;
border-radius: 50%;
display: inline-block;
margin-right: 5px;
}
These animations provide visual feedback that guides users through the application:
- The pulsing glow on the core essence position draws attention to the center of the matrix
- Card animations highlight active interpretations
- Smooth transitions reveal results after calculations
These subtle touches significantly enhance the perceived quality and polish of the application.
Conclusion
The Matrix of Destiny application demonstrates how to combine ancient numerological concepts with modern web technologies. By using SVG for visualization, we’ve created an interactive tool that brings numerology to life in a visually engaging way.
The combination of precise calculations, thoughtful visual design, and meaningful interactions creates an application that’s both functional and aesthetically pleasing. Users can explore their numerological matrix, discovering connections between different aspects of their life and gaining insights from the interpretations provided.
In the next part of our series, we’ll expand the application’s capabilities by:
- Adding chart comparisons between different birth dates
- Implementing progressed matrices that show how numerological influences change over time
- Creating a report generator that provides detailed interpretations in a downloadable format
- Enhancing the visual experience with advanced SVG filters and effects
You can explore the complete code for this project in our GitHub repository: Matrix of Destiny Project
Whether you’re a developer interested in SVG visualization techniques or a numerology enthusiast eager to explore your personal matrix, we hope this project inspires you to blend ancient wisdom with modern technology in creative ways.