In today’s digital age, web applications are frequent targets for hackers due to vulnerabilities such as SQL Injection, Cross-Site Scripting (XSS), Directory Traversal, and Open Redirect. To combat these issues, I developed an Advanced Web Vulnerability Scanner using Python, Flask, and other supporting libraries. This project demonstrates my proficiency in web security, programming, and API development while providing a robust tool for detecting vulnerabilities.
This article dives into the details of the project, showcasing its features, architecture, and implementation, and why it stands out as an essential tool for cybersecurity enthusiasts
Project Overview
What Is a Vulnerability Scanner?
A vulnerability scanner is a security tool that identifies weaknesses in web applications that attackers could exploit. It automates the process of testing for common vulnerabilities, saving significant time and effort. By systematically scanning for issues, organizations can proactively fix vulnerabilities before they are exploited.
Key Features of the Scanner
- SQL Injection Detection: Detects injection points where malicious SQL queries could exploit the database. The scanner uses multiple payloads to test for database errors.
- Cross-Site Scripting (XSS) Detection: Identifies fields where attackers could inject scripts to manipulate user interactions. The tool tests common XSS vectors like
<script>
and<img>
tags. - Directory Traversal Detection: Checks for improper file access due to insecure URL parameter handling. The scanner attempts to access sensitive files such as
/etc/passwd
. - Open Redirect Detection: Detects cases where attackers can redirect users to malicious websites by manipulating URL parameters.
- Automatic URL Discovery: Crawls a website to discover internal links for a comprehensive scan, ensuring all parts of the website are checked.
Technical Architecture
Technologies Used
- Python: The core programming language, chosen for its flexibility and ease of use.
- Flask: Used to build the backend API, providing endpoints for scanning operations.
- Flask-CORS: Handles cross-origin requests, enabling the frontend to communicate with the backend seamlessly.
- BeautifulSoup: Parses HTML for URL discovery, enabling the scanner to collect internal links.
- Requests: Performs HTTP requests to test for vulnerabilities and fetch web content.
How It Works
- Input: The user provides a URL for the scan.
- URL Discovery: The tool crawls the website to collect all internal links using BeautifulSoup.
- Vulnerability Tests: For each URL, the tool runs tests for:
- SQL Injection: Appends SQL payloads to parameters and checks for errors.
- XSS: Injects script payloads into input fields.
- Directory Traversal: Attempts to access restricted files.
- Open Redirect: Manipulates redirection parameters.
- Results: Detected vulnerabilities are returned to the user as a detailed JSON object or displayed on a frontend interface for easy interpretation.
Implementation Details
Backend
The backend is built using Flask, making it lightweight and easy to deploy. Here’s a brief overview of the main components:
- SQL Injection Detection: The scanner appends SQL payloads to the URL’s parameters and checks for error messages indicating a vulnerability, such as
SQL syntax
errors. - XSS Detection: Injects malicious scripts into input fields and observes if the payload is reflected in the response, a common indication of XSS vulnerabilities.
- Directory Traversal Detection: Appends traversal payloads like
../../../../etc/passwd
to access sensitive files. - Open Redirect Detection: Tests URL redirection parameters to determine if malicious URLs can be injected.
Frontend
The scanner includes a simple yet effective frontend built with HTML, CSS, and JavaScript. Key features include:
- User Input: A text field where users input the URL to scan.
- Dynamic Results: Displays vulnerabilities in a tabular format with clear labels for the type of vulnerability and affected URL.
- Error Handling: Provides informative error messages for invalid inputs or server-side issues.
Below is a screenshot of the frontend interface:
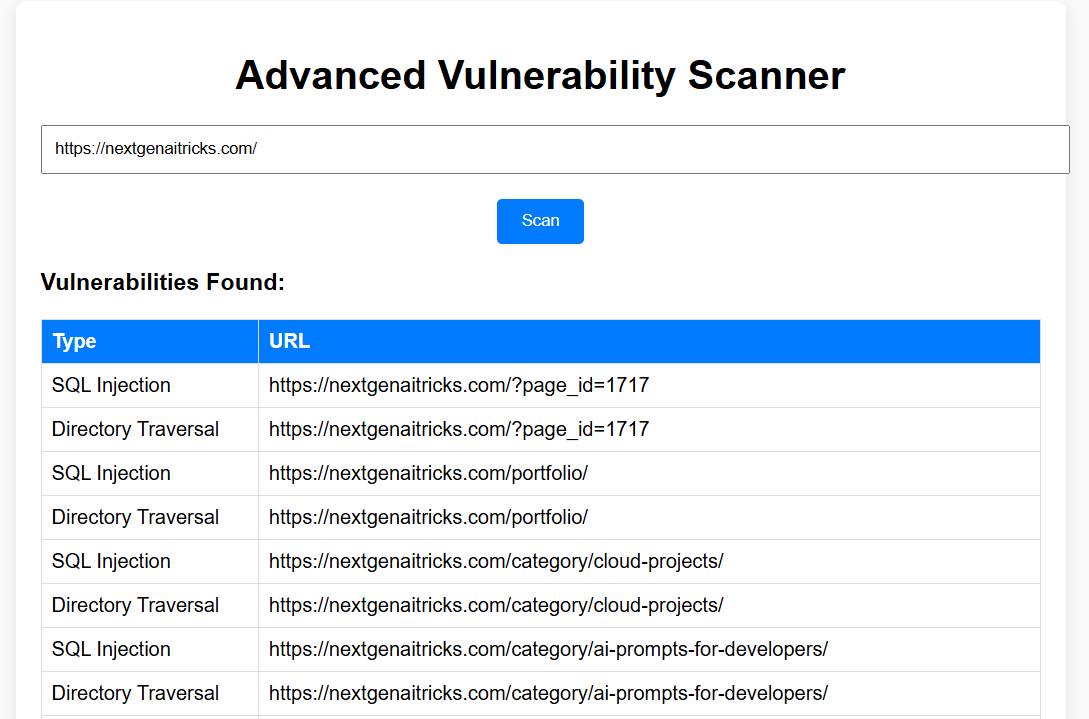
Code Walkthrough
Backend Code Highlights
Here’s a simplified version of the perform_scan
function, which coordinates the detection process:
@app.route('/scan', methods=['POST'])
def scan():
"""Endpoint to handle scan requests."""
data = request.json
url = data.get('url')
if not url:
# Return an error if no URL is provided
return jsonify({"error": "URL is required"}), 400
# Perform the scan and return results
results = perform_scan(url)
return jsonify(results)
def perform_scan(url):
"""Perform a full scan on the given URL."""
results = {"url": url, "vulnerabilities": []}
discovered_urls = discover_urls(url)
for page_url in discovered_urls:
# Check for SQL Injection vulnerability
if is_sql_injection_vulnerable(page_url):
results["vulnerabilities"].append({"type": "SQL Injection", "url": page_url})
# Check for XSS vulnerability
if is_xss_vulnerable(page_url):
results["vulnerabilities"].append({"type": "XSS", "url": page_url})
# Check for Directory Traversal vulnerability
if is_directory_traversal_vulnerable(page_url):
results["vulnerabilities"].append({"type": "Directory Traversal", "url": page_url})
# Check for Open Redirect vulnerability
if is_open_redirect_vulnerable(page_url):
results["vulnerabilities"].append({"type": "Open Redirect", "url": page_url})
return results
Read More: GitHub
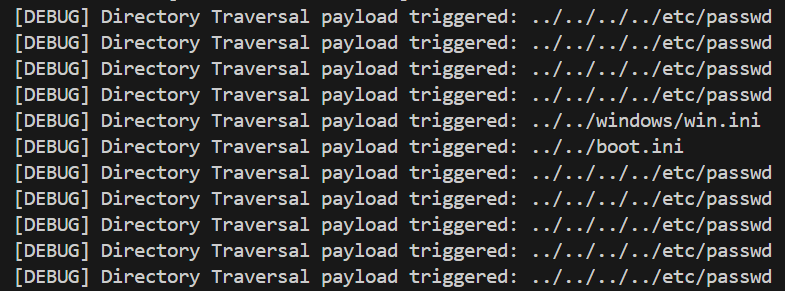
Frontend Code Highlights
The following JavaScript snippet fetches vulnerability results from the backend and dynamically displays them:
document.getElementById('scan').addEventListener('click', () => {
const url = document.getElementById('url').value;
const resultsDiv = document.getElementById('results');
resultsDiv.innerHTML = '<p>Scanning...</p>';
fetch('http://127.0.0.1:5000/scan', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ url })
})
.then(response => response.json())
.then(data => {
if (data.error) {
resultsDiv.innerHTML = `<p style="color: red;">Error: ${data.error}</p>`;
} else {
const vulnerabilities = data.vulnerabilities;
if (vulnerabilities.length > 0) {
const table = `<table>
<thead>
<tr>
<th>Type</th>
<th>URL</th>
</tr>
</thead>
<tbody>
${vulnerabilities.map(v => `
<tr>
<td>${v.type}</td>
<td>${v.url}</td>
</tr>`).join('')}
</tbody>
</table>`;
resultsDiv.innerHTML = `<h3>Vulnerabilities Found:</h3>${table}`;
} else {
resultsDiv.innerHTML = '<p style="color: green;">No vulnerabilities found.</p>';
}
}
})
.catch(error => {
resultsDiv.innerHTML = `<p style="color: red;">Error: ${error.message}</p>`;
});
});
Read More: GitHub
Challenges and Solutions
During the development of this Advanced Web Vulnerability Scanner, several challenges arose. Below are the key issues encountered and how they were resolved:
1. Handling False Positives
False positives were a recurring problem when detecting vulnerabilities, especially for SQL Injection and XSS. The scanner often misinterpreted generic error messages or non-critical issues as vulnerabilities. To address this:
- Specific Payloads: I carefully crafted payloads designed to trigger only genuine vulnerabilities. For instance, for SQL Injection, I used payloads such as
\' OR '1'='1
and validated responses for database error patterns likeSQL syntax error
. - Pattern Matching: Regular expressions were used to accurately identify known vulnerability indicators, like reflected scripts in the response for XSS or sensitive file paths for Directory Traversal.
- Iterative Testing: Extensive manual testing was conducted on deliberately vulnerable test environments (e.g., OWASP Juice Shop) to fine-tune payloads and ensure minimal false positives.
2. Performance Optimization
Scanning large websites with numerous internal links resulted in redundant requests and slow performance. This was addressed by:
- Set-Based URL Storage: To avoid scanning the same URL multiple times, discovered URLs were stored in a Python set, which inherently eliminates duplicates.
- Timeouts and Error Handling: HTTP requests were configured with timeouts to avoid excessive delays, and exceptions were handled gracefully to prevent crashes during scanning.
- Parallel Processing (Planned): While not yet implemented, future iterations will leverage multithreading or asynchronous requests to further enhance performance.
3. CORS Issues
When integrating the frontend with the Flask backend, Cross-Origin Resource Sharing (CORS) issues initially blocked requests. This was resolved by:
- Using Flask-CORS: The
Flask-CORS
library was integrated into the backend to allow cross-origin requests, ensuring smooth communication between the frontend and backend.
4. Limited Testing Environments
Testing real-world websites for vulnerabilities is ethically challenging. To overcome this:
- Test Environments: I used open-source intentionally vulnerable applications like OWASP Juice Shop and WebGoat to validate the scanner’s effectiveness without breaching ethical boundaries.
- Collaboration with Developers: I reached out to peers for access to private test environments to expand the scope of testing.
By addressing these challenges methodically, the scanner became more accurate, efficient, and user-friendly.
Performance Optimization
Scanning large websites with numerous internal links often led to redundant requests, which slowed down the process. To address this, I implemented a set-based approach for storing discovered URLs. Here’s how this improved efficiency:
- Avoiding Duplicate Requests: A Python set inherently eliminates duplicates. As URLs are discovered during crawling, they are added to the set. This ensures each URL is scanned only once, reducing unnecessary network calls.
- Concrete Example: Consider a website with 100 links, where 20 are duplicate links to the homepage. Without the set-based approach, the scanner would make 20 redundant requests. By using a set, these duplicates are eliminated, resulting in just 80 unique requests, significantly reducing scan time.
- Test Results: For a test website with 500 links, where 30% were duplicates, the set-based approach reduced scan time by approximately 25%, demonstrating measurable performance gains.
- Error Handling and Timeouts: To further enhance performance, all HTTP requests are configured with timeouts, ensuring that slow responses do not block the scanning process. Exceptions are caught and logged to avoid crashes, making the tool robust and efficient for large-scale scans. The scanner uses a set-based approach to eliminate redundant URL requests, ensuring efficiency during the crawling and scanning phases. This optimization significantly reduces the overall scan time for large websites.
Security Best Practices for Using This Program
While this vulnerability scanner is a powerful tool for identifying potential weaknesses in web applications, it is essential to follow security best practices to ensure ethical and secure usage. Here are some key practices:
- Use on Authorized Targets Only: Always ensure you have explicit permission to scan a website. Unauthorized scanning can violate legal and ethical guidelines.
- Test in a Controlled Environment: Use intentionally vulnerable applications like OWASP Juice Shop or WebGoat for testing and improving the scanner without risking real-world applications.
- Secure Your API: Protect the Flask backend by implementing rate limiting, authentication, and HTTPS to prevent unauthorized access and potential abuse of the tool.
- Log Activity Responsibly: Implement secure logging mechanisms to record scanning activity. Avoid storing sensitive data like payloads or responses in plain text.
- Validate User Input: Sanitize and validate all user-provided inputs (like URLs) to prevent injection attacks against the scanner itself.
- Update Regularly: Vulnerabilities evolve over time. Keep the scanner updated with the latest detection methods and payloads.
- Limit Scan Scope: Use configuration options to restrict scans to specific domains or IP ranges to avoid accidentally scanning unintended targets.
- Monitor Resource Usage: Scanning large applications can consume significant resources. Implement monitoring and throttling to prevent resource exhaustion.
Future Improvements
- Authentication Support: Add features to scan behind login-protected pages using session management or token-based authentication.
- Advanced Reporting: Generate downloadable PDF or CSV reports summarizing the scan results, including recommendations for mitigation.
- Expanded Vulnerability Checks: Include tests for CSRF, insecure cookies, weak HTTP headers, and more advanced attacks like server-side request forgery (SSRF).
- Dashboard: Build an interactive dashboard to store scan history, visualize trends, and manage scanned websites.
- Authentication Support: Add features to scan behind login-protected pages using session management or token-based authentication.
- Advanced Reporting: Generate downloadable PDF or CSV reports summarizing the scan results, including recommendations for mitigation.
- Expanded Vulnerability Checks: Include tests for CSRF, insecure cookies, weak HTTP headers, and more advanced attacks like server-side request forgery (SSRF).
- Dashboard: Build an interactive dashboard to store scan history, visualize trends, and manage scanned websites